Thermodynamic processes calculator is a simple application that only counts thermodynamic changes of humid air in some cases but it still needed SQL or CSV table to finish calculations. In calculations of a point after cooling process the application needs a table where it can find temperature regard vapor pressure with that data:
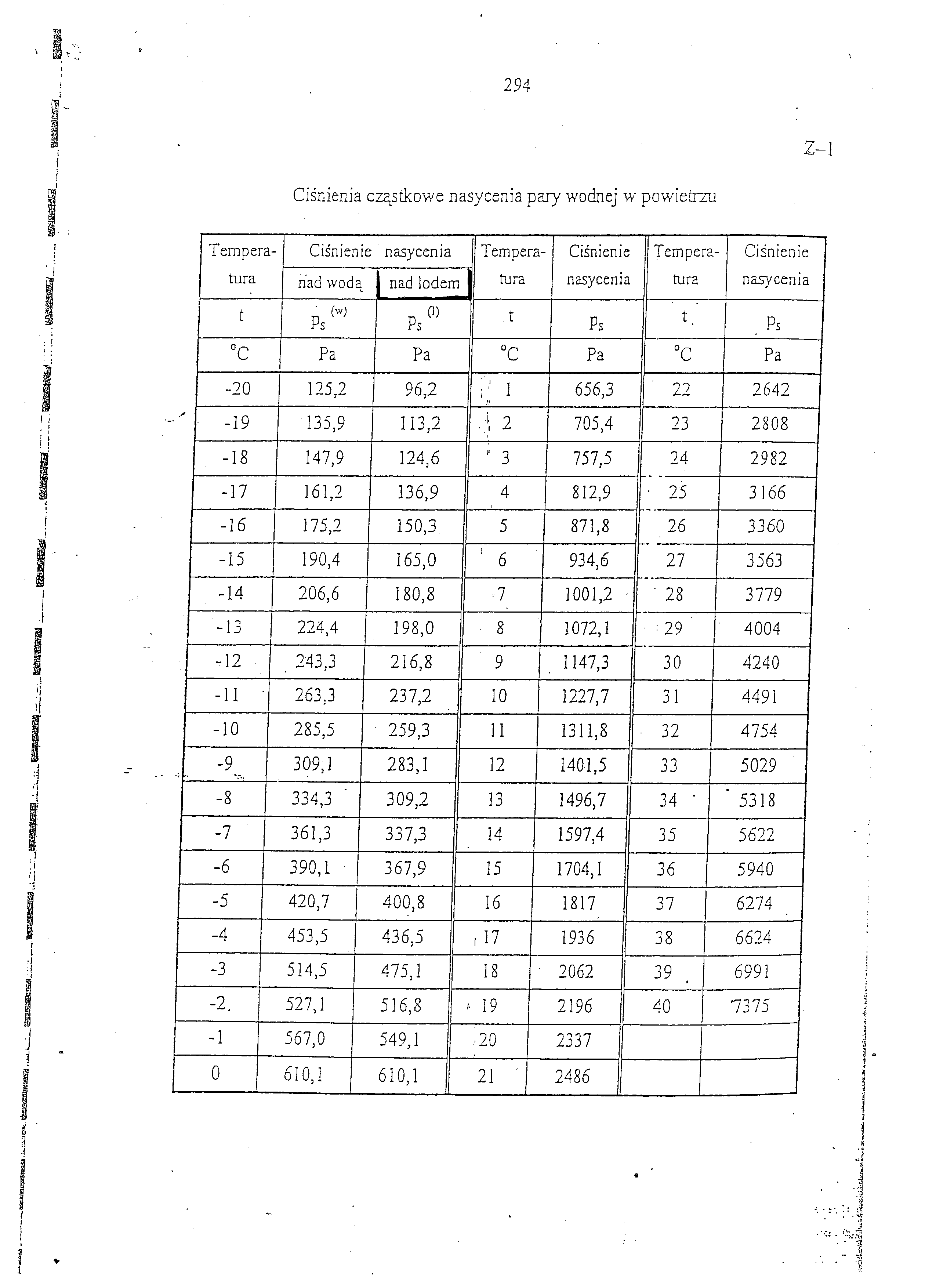
I assumed that it is the best if I use SQLite to accomplish that. That SQL implementation doesn’t need any server application, it uses only one file. I prepared the file with SQLite DB Browser.
The hardest task came after that. How to implement SQLite query in my application despite I am completely new in SQLite. I made some research and I found some useful libraries to do that. In my opinion the best option is SQLiteC++ library. It is complete object objected code with exceptions handling. Unfortunately I had some problems with implementing it in my application, but this is normal 🙂 I solve these problems with help of uncle GOOGlE :).
I wanted it to be useful on GNU/Linux and on Windows, unfortunately I don’t know yet how to make DLLs so I just added the files to building tree of my application. Also with sqlite3 source files. I bundled all files in to one folder and Then I changed all includes as local:
#include <SQLiteCpp/Assertion.h> #include <SQLiteCpp/Exception.h> #include <SQLiteCpp/Database.h> #include <SQLiteCpp/Statement.h> #include <SQLiteCpp/Column.h> #include <SQLiteCpp/Transaction.h>
for
#include "assertion.h" #include "exception.h" #include "database.h" #include "statement.h" #include "column.h" #include "transaction.h"
That is example only from file SQLiteCpp.h, I had to change like this all files – headers and source files.
Also I added in .pro file one line:
unix:!macx: LIBS += -ldl
And it can be compiled under GNU/Linux and under Windows 10 as well.
Then I changed a little bit sample query from the project github site like this:
try { // Open a database file SQLite::Database db("temperature.db"); // Compile a SQL query, containing one parameter (index 1) SQLite::Statement query(db, "SELECT temperature FROM temperature ORDER BY ABS(? - vapor) LIMIT 1"); // Bind the integer value 6 to the first parameter of the SQL query query.bind(1, equilibriumVaporPoint); // Loop to execute the query step by step, to get rows of result query.executeStep(); temperature = query.getColumn(0); } catch (std::exception& e) { std::cerr << "exception: " << e.what() << std::endl; }